Summary: in this tutorial, you’ll learn how to use the Tkinter Text widget to add a text editor to your application.
Introduction to the Tkinter Text widget #
The Text
widget allows you to display and edit multi-line textarea with various styles. Besides the plain text, the Text
widget supports embedded images and links.
To create a text widget, you use the following syntax:
text = tk.Text(master, conf={}, **kw)
In this syntax:
- The
master
is the parent component of theText
widget. - The
cnf
is a dictionary that specifies the widget’s configuration. - The
kw
is one or more keyword arguments used to configure theText
widget.
Note that the Text
widget is only available in the Tkinter module, not the Tkinter.ttk
module.
The following example creates a Text
widget with eight rows and places it on the root window:
from tkinter import Tk, Text
root = Tk()
root.resizable(False, False)
root.title("Text Widget Example")
text = Text(root, height=8)
text.pack()
root.mainloop()
Code language: JavaScript (javascript)
Output:
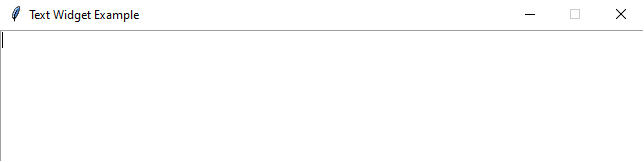
In this example, the height
argument specifies the number of rows of the Text
widget.
Inserting initial content #
To insert contents into the text area, you use the insert()
method. For example:
from tkinter import Tk, Text
root = Tk()
root.resizable(False, False)
root.title("Text Widget Example")
text = Text(root, height=8)
text.pack()
text.insert('1.0', 'This is a Text widget demo')
root.mainloop()
Code language: JavaScript (javascript)
Output:
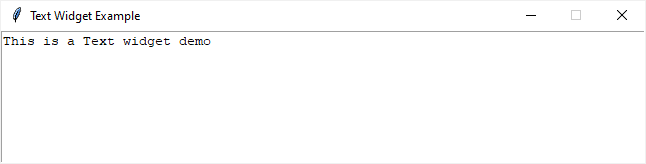
The first argument of the insert()
method is the position where you want to insert the text.
The position has the following format:
'line.column'
Code language: JavaScript (javascript)
In the above example, '1.0'
means line 1, character 0, which is the first character of the first line on the text area.
Retrieving the text value #
To retrieve the contents of a Text
widget, you use its get()
method. For example:
text_content = text.get('1.0','end')
Code language: JavaScript (javascript)
The get()
method accepts two arguments. The first argument is the start position, and the second is the end position.
To retrieve only part of the text, you can specify different start and end positions.
Disabling the Text widget #
To prevent users from changing the contents of a Text
widget, you can disable it by setting the state
option to 'disabled'
like this:
text['state'] = 'disabled'
Code language: JavaScript (javascript)
To re-enable editing, you can change the state
option back to normal
:
text['state'] = 'normal'
Code language: JavaScript (javascript)
Summary #
- Use Tkinter
Text
widget to create a multi-line text area.