Summary: in this tutorial, you’ll learn about the Tkinter StringVar
object and how to use it to manipulate values of widgets.
Introduction to the Tkinter StringVar
The Tkinter StringVar
helps you manage the value of a widget such as a Label
or Entry
more effectively.
To create a new StringVar
object, you use the StringVar
constructor like this:
string_var = tk.StringVar(container, value, name)
Code language: Python (python)
The StringVar
constructor accepts three optional arguments:
container
is a widget that theStringVar
object associated with. If you skip the container, it defaults to the root window.value
is the initial value that defaults to an empty string''
.name
is a Tcl name that defaults toPY_VARnum
e.g.PY_VAR1
,PY_VAR2
, etc.
After creating the StringVar
object, you can assign it to the textvariable
of a widget that accepts a StringVar
object.
For example, the following assigns the string_var
to textvariable
of the Entry
widget:
name_entry = ttk.Entry(root, textvariable=string_var)
Code language: Python (python)
To get the current value of the Entry
widget, you can use the get()
method of the StringVar
object:
name_var.get()
Code language: Python (python)
The StringVar
object will notify you whenever its value changes. This feature is useful if you want to automatically update other widgets based on the current value of the StringVar
object.
To invoke a callback whenever the value of an StringVar
object changes, you use the trace()
method of the StringVar
object:
string_var.trace('w', callback)
Code language: Python (python)
The 'w'
mode will automatically invoke the callback
whenever the value of the string_var
changes.
The StringVar
also provides you with two other modes 'r'
and 'u'
:
'r'
(read) – invoke the callback whenever the variable is read.'u'
(unset) – invoke the callback whenever the variable is deleted.
Tkinter StringVar example
The following example illustrates how to use the StringVar
object for an Entry
widget:
import tkinter as tk
from tkinter import ttk
class App(tk.Tk):
def __init__(self):
super().__init__()
self.title('Tkinter StringVar')
self.geometry("300x80")
self.name_var = tk.StringVar()
self.columnconfigure(0, weight=1)
self.columnconfigure(1, weight=1)
self.columnconfigure(2, weight=1)
self.create_widgets()
def create_widgets(self):
padding = {'padx': 5, 'pady': 5}
# label
ttk.Label(self, text='Name:').grid(column=0, row=0, **padding)
# Entry
name_entry = ttk.Entry(self, textvariable=self.name_var)
name_entry.grid(column=1, row=0, **padding)
name_entry.focus()
# Button
submit_button = ttk.Button(self, text='Submit', command=self.submit)
submit_button.grid(column=2, row=0, **padding)
# Output label
self.output_label = ttk.Label(self)
self.output_label.grid(column=0, row=1, columnspan=3, **padding)
def submit(self):
self.output_label.config(text=self.name_var.get())
if __name__ == "__main__":
app = App()
app.mainloop()
Code language: Python (python)
Output:
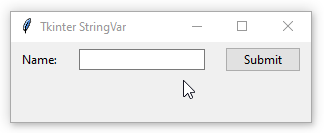
How it works.
First, create a new StringVar
object in the __init__()
method of the App
class:
self.name_var = tk.StringVar()
Code language: Python (python)
Second, assign the StringVar
object to the textvariable
option of the Entry
widget in the create_widgets()
method:
name_entry = ttk.Entry(self, textvariable=self.name_var)
Code language: Python (python)
Third, set the text of the output_label
widget to the value of the name_var
object when the button is clicked.
self.output_label.config(text=self.name_var.get())
Code language: Python (python)
Tkinter StringVar – Tracing text changes example
The following example illustrates how to use the StringVar
object to trace text changes.
The root window has two Entry
widgets: password and confirm password. If you enter the confirm password that is different from the password, it’ll show an error message. Otherwise, it’ll show a success message:
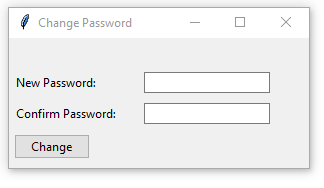
import tkinter as tk
from tkinter import ttk
class App(tk.Tk):
ERROR = 'Error.TLabel'
SUCCESS = 'Success.TLabel'
def __init__(self):
super().__init__()
self.title('Change Password')
self.geometry("300x130")
self.password_var = tk.StringVar()
self.confirm_password_var = tk.StringVar()
self.confirm_password_var.trace('w', self.validate)
self.columnconfigure(0, weight=1)
self.columnconfigure(1, weight=1)
self.columnconfigure(2, weight=1)
# set style
self.style = ttk.Style(self)
self.style.configure('Error.TLabel', foreground='red')
self.style.configure('Success.TLabel', foreground='green')
self.create_widgets()
def create_widgets(self):
""" create a widget
"""
padding = {'padx': 5, 'pady': 5, 'sticky': tk.W}
# message
self.message_label = ttk.Label(self)
self.message_label.grid(column=0, row=0, columnspan=3, **padding)
# password
ttk.Label(self, text='New Password:').grid(column=0, row=1, **padding)
password_entry = ttk.Entry(
self, textvariable=self.password_var, show='*')
password_entry.grid(column=1, row=1, **padding)
password_entry.focus()
# Confirm password
ttk.Label(self, text='Confirm Password:').grid(
column=0, row=2, **padding)
confirm_password = ttk.Entry(
self, textvariable=self.confirm_password_var, show='*')
confirm_password.grid(column=1, row=2, **padding)
confirm_password.focus()
# Change button
submit_button = ttk.Button(self, text='Change')
submit_button.grid(column=0, row=3, **padding)
def set_message(self, message, type=None):
""" set the error or success message
"""
self.message_label['text'] = message
if type:
self.message_label['style'] = type
def validate(self, *args):
""" validate the password
"""
password = self.password_var.get()
confirm_password = self.confirm_password_var.get()
if confirm_password == password:
self.set_message(
"Success: The new password looks good!", self.SUCCESS)
return
if password.startswith(confirm_password):
self.set_message('Warning: Keep entering the password')
self.set_message("Error: Passwords don't match!", self.SUCCESS)
if __name__ == "__main__":
app = App()
app.mainloop()
Code language: Python (python)
How it works:
First, define two constants ERROR
and SUCCESS
that will be set to the message_label
based on the result of the validation:
ERROR = 'Error.TLabel'
SUCCESS = 'Success.TLabel'
WARNING = 'Warning.TLabel'
Code language: Python (python)
Second, create two StringVar
objects:
self.password_var = tk.StringVar()
self.confirm_password_var = tk.StringVar()
Code language: Python (python)
Third, use the trace()
method to call the self.validate()
method whenever the text of the password confirmation widget changes:
self.confirm_password_var.trace('w', self.validate)
Code language: Python (python)
Finally, show the success message if the passwords match in the validate()
method. Otherwise, show a warning message if the password starts with the confirmed password. If the passwords don’t match, show an error message.
Summary
- Use Tkinter
StringVar
object to manage values of widgets more effectively. - Assign the
StringVar
object to thetextvariable
of the widget. - Use the
trace()
method of theStringVar
object to trace the text changes.