Summary: in this tutorial, you’ll learn how to set options for a Tk themed widget using the widget constructor, a dictionary index, and config()
method.
When working with themed widgets, you often need to set their attributes such as text and image.
Tkinter allows you to set the options of a widget using one of the following ways:
- Use the widget constructor during the widget’s creation.
- Set a property value using a dictionary index after creating the widget.
- Call the
config()
method with keyword arguments.
1) Using the widget constructor when creating the widget
The following illustrates how to use the widget constructor to set the text
option for the Label
widget:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
ttk.Label(root, text='Hi, there').pack()
root.mainloop()
Code language: JavaScript (javascript)
Output:
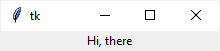
2) Using a dictionary index after widget creation
The following program shows the same label but uses a dictionary index to set the text
option for the Label
widget:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
label = ttk.Label(root)
label['text'] = 'Hi, there'
label.pack()
root.mainloop()
Code language: JavaScript (javascript)
The following code sets the text
options for the label:
label['text'] = 'Hi, there'
Code language: JavaScript (javascript)
3) Using the config() method with keyword arguments
The following program illustrates how to use the config()
method with a keyword argument to set the text
option for the label:
import tkinter as tk
from tkinter import ttk
root = tk.Tk()
label = ttk.Label(root)
label.config(text='Hi, there')
label.pack()
root.mainloop()
Code language: JavaScript (javascript)
Summary
Ttk widgets offer three ways to set their options:
- Use the widget constructor during the widget’s creation.
- Set a property value using a dictionary index after creating the widget.
- Call the
config()
method with keyword arguments.