Summary: in this tutorial, you’ll learn about the Tkinter Checkbox widget and how to use it effectively.
Introduction to the Tkinter checkbox widget #
A checkbox is a widget that allows you to check and uncheck. A checkbox can hold a value and invoke a function automatically when its state changes.
Typically, you use a checkbox when you want to ask users to choose between two values.
To create a checkbox, you use the ttk.Checkbutton
constructor:
checkbox = ttk.Checkbutton(
master,
text='<checkbox label>',
command=callback,
variable=variable,
onvalue='<value_when_checked>',
offvalue='<value_when_unchecked>'
)
Code language: Python (python)
In this syntax:
- The
master
argument specifies the master widget that you want to place the checkbox. - The
text
argument specifies the label for the checkbox. - The
command
is a callable that will be called once the checkbox is checked or unchecked. - The
variable
holds the current value of the checkbox. Typically, you use a BooleanVar object to track whether the checkbox is checked or not. If you want other values thanTrue
andFalse
, you can specify them in theonvalue
andoffvalue
options.
Tkinter checkbox example #
The following program illustrates how to use a checkbox widget. Once you check or uncheck the checkbox, a message box will show the on value and the off value accordingly:
import tkinter as tk
from tkinter import ttk
from tkinter.messagebox import showinfo
root = tk.Tk()
root.geometry('300x200')
root.title('Checkbox Demo')
def show_message():
showinfo(
title='Result',
message='You agreed.' if agreement_var.get() else 'You did not agree.'
)
agreement_var = tk.BooleanVar()
checkbox = ttk.Checkbutton(
root,
text='I agree',
command=show_message,
variable=agreement_var
)
checkbox.pack()
root.mainloop()
Code language: Python (python)
Output:
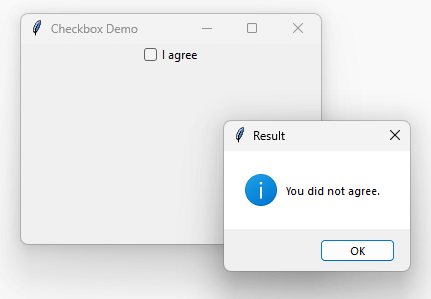
How it works.
First, create a BooleanVar object that will hold the the state of the checkbox:
agreement_var = tk.BooleanVar()
Second, define a function that will execute when the state of the checkbox changes. The function shows a message whether you check or uncheck the checkbox:
def show_message():
showinfo(
title='Result',
message='You agreed.' if agreement_var.get() else 'You did not agree.'
)
Code language: JavaScript (javascript)
Third, create a checkbox widget and set its options accordingly:
checkbox = ttk.Checkbutton(
root,
text='I agree',
command=show_message,
variable=agreement_var
)
Code language: JavaScript (javascript)
Summary #
- Use
Checkbutton()
to create a checkbox. - Use
command
argument to specify a function that executes when the button is checked or unchecked. - Use the
onvalue
andoffvalue
to determine what value thevariable
will take.