Summary: in this tutorial, you’ll learn how to use the Tkinter askokcancel()
function to show a confirmation dialog.
Introduction to the Tkinter askokcancel() function #
The askokcancel()
function shows a confirmation dialog that has two buttons: OK
and Cancel
.
answer = askokcancel(title, message, **options)
Code language: Python (python)
If you click the OK
button, the function returns True
. However, if you click the Cancel
button, the function returns False
.
Tkinter askokcancel() example #
The following program shows a Delete All button. If you click the button, the program will show a confirmation dialog that has two buttons: OK
and Cancel
.
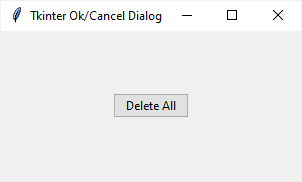
If you click the OK
button, the program will show a message box indicating that all the data has been deleted successfully:
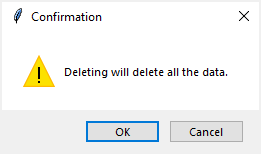
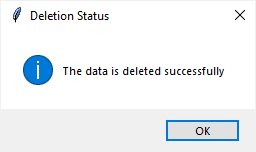
The program:
import tkinter as tk
from tkinter import ttk
from tkinter.messagebox import askokcancel, showinfo, WARNING
# create the root window
root = tk.Tk()
root.title('Tkinter Ok/Cancel Dialog')
root.geometry('300x150')
# click event handler
def confirm():
answer = askokcancel(
title='Confirmation',
message='Deleting will delete all the data.',
icon=WARNING)
if answer:
showinfo(
title='Deletion Status',
message='The data is deleted successfully')
ttk.Button(
root,
text='Delete All',
command=confirm).pack(expand=True)
# start the app
root.mainloop()
Code language: Python (python)
The following program does the same as the program above but use the object-oriented programming approach:
import tkinter as tk
from tkinter import ttk
from tkinter.messagebox import askokcancel, showinfo, WARNING
class App(tk.Tk):
def __init__(self):
super().__init__()
self.title('Tkinter Ok/Cancel Dialog')
self.geometry('300x150')
delete_button = ttk.Button(
self,
text='Delete All',
command=self.confirm)
delete_button.pack(expand=True)
def confirm(self):
answer = askokcancel(
title='Confirmation',
message='Deleting will delete all the data.',
icon=WARNING)
if answer:
showinfo(
title='Deletion Status',
message='The data is deleted successfully')
if __name__ == "__main__":
app = App()
app.mainloop()
Code language: Python (python)
Summary #
- Use the Tkinter
askokcancel()
function to display a confirmation dialog with two buttonsOK
andCancel
. - The
askokcancel()
function returnsTrue
if you click theOK
button andFalse
if you click theCancel
button.