Summary: in this tutorial, you’ll learn about the Python try...except...finally
statement.
Introduction to Python try…catch…finally statement
The try...except
statement allows you to catch one or more exceptions in the try
clause and handle each of them in the except
clauses.
The try...except
statement also has an optional clause called finally
:
try:
# code that may cause exceptions
except:
# code that handle exceptions
finally:
# code that clean up
Code language: PHP (php)
The finally
clause always executes whether an exception occurs or not. And it executes after the try
clause and any except
clause.
The following flowchart illustrates the try...catch...finally
clause:
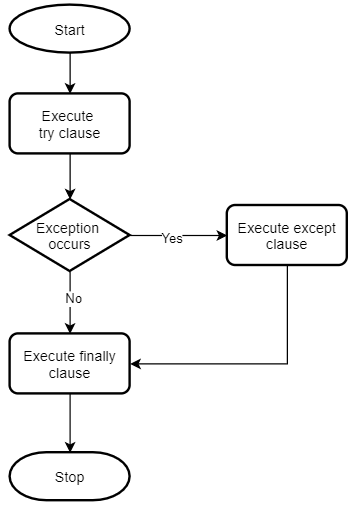
Python try…catch…finally statement examples
The following example uses the try...catch...finally
statement:
a = 10
b = 0
try:
c = a / b
print(c)
except ZeroDivisionError as error:
print(error)
finally:
print('Finishing up.')
Code language: PHP (php)
Output:
division by zero
Finishing up.
In this example, the try
clause causes a ZeroDivisionError
exception both except
and finally
clause executes.
The try
clause in the following example doesn’t cause an error. Therefore, all statements in the try
and finally
clauses execute:
a = 10
b = 2
try:
c = a / b
print(c)
except ZeroDivisionError as error:
print(error)
finally:
print('Finishing up.')
Code language: PHP (php)
Output:
5.0
Finishing up.
Code language: CSS (css)
Python try…finally statement
The catch
clause in the try...catch...finally
statement is optional. So you can write it like this:
try:
# the code that may cause an exception
finally:
# the code that always executes
Code language: PHP (php)
Typically, you use this statement when you cannot handle the exception but you want to clean up resources. For example, you want to close the file that has been opened.
Summary
- Use Python
try...catch...finally
statement to execute a code block whether an exception occurs or not. - Use the
finally
clause to clean up the resources such as closing files.