Summary: in this tutorial, you’ll learn how to create a time entry widget using the PyQt QTimeEdit
class.
Introduction to the PyQt QTimeEdit widget
The QTimeEdit
class allows you to create a widget for editing time:
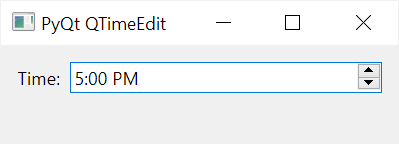
The QTimeEdit
widget allows you to edit the time using the keyboard or up/down arrow keys to increase/decrease the time value. Also, you can use the left/right arrow key to move between the day, month, and year sections within the entry.
The QTimeEdit
has the following useful properties:
Property | Description |
---|---|
time() | Return the time displayed by the widget. The return value has the type of . To convert it to a Python datetime.time object, you can use the toPyTime() method of the class. |
minimumTime | Specify the earliest time that can be set by the user. |
maximumTime | Specify the latest time that can be set by the user. |
displayFormat | is a string that formats the time displayed in the widget. |
The QTimeEdit
emits the editingFinished
signal when the editing is finished.
PyQt QTimeEdit widget example
The following program uses the QTimeEdit
class to create a widget for editing time:
import sys
from PyQt6.QtWidgets import QApplication, QWidget, QTimeEdit, QLabel, QFormLayout
class MainWindow(QWidget):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.setWindowTitle('PyQt QTimeEdit')
self.setMinimumWidth(200)
# create a grid layout
layout = QFormLayout()
self.setLayout(layout)
self.time_edit = QTimeEdit(self)
self.time_edit.editingFinished.connect(self.update)
self.result_label = QLabel('', self)
layout.addRow('Time:', self.time_edit)
layout.addRow(self.result_label)
# show the window
self.show()
def update(self):
value = self.time_edit.time()
self.result_label.setText(str(value.toPyTime()))
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
sys.exit(app.exec())
Code language: Python (python)
How it works.
First, create the QTimeEdit
widget:
self.time_edit = QTimeEdit(self)
Code language: Python (python)
Second, connect the editingFinished()
signal to the update()
method:
self.time_edit.editingFinished.connect(self.update)
Code language: Python (python)
Third, create a QLabel
widget to display the value of the time_edit
widget once the editing is finished:
self.result_label = QLabel('', self)
Code language: Python (python)
Finally, define the update()
method that updates the label widget with the current value of the time entry:
def update(self):
value = self.time_edit.time()
self.result_label.setText(str(value.toPyTime()))
Code language: Python (python)
Output:
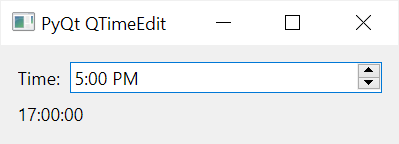
Summary
- Use the
QTimeEdit
class to create a date entry widget. - Use the
date()
method to get the current value of theQTimeEdit
widget. - Connect to the
editingFinished
signal to trigger an action when editing is finished.