Summary: in this tutorial, you’ll learn how to create a date & time entry widget using the PyQt
class.QDateTimeEdit
Introduction to the PyQt QDateTimeEdit widget
The
class allows you to create a widget for editing dates & times:QDateTimeEdit
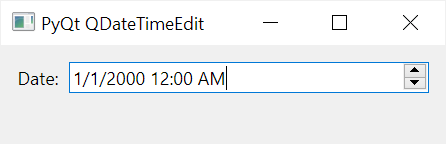
The
widget allows you to edit the date and time using the keyboard or up/down arrow keys to increase/decrease the value.QDateTimeEdit
Also, you can use the left/right arrow key to move between the day, month, year, hour, and minute sections of the entry.
The
has the following useful methods and properties:QDateTimeEdit
Property | Description |
---|---|
date() | Return the date value displayed by the widget. The return type is . To convert it to a datetime.date object, you use the toPyDate() method of the class. |
time() | Return the time displayed by the widget. The return value has the type of . Use the toPyTime() method to convert it to a Python datetime.time object. |
dateTime() | Return the date and time value displayed by the widget. The return type is . |
minimumDate | The earliest date that can be set by the user. |
maximumDate | The latest date that can be set by the user. |
minimumTime | The earliest time that can be set by the user. |
maximumTime | The latest time that can be set by the user. |
minimumDateTime | The earliest date & time that can be set by the user. |
maximumDateTime | The latest date & time that can be set by the user. |
calendarPopup | Display a calendar popup if it is True. |
displayFormat | is a string that formats the date displayed in the widget. |
The
emits the QDateTimeEdit
editingFinished()
signal when the editing is finished. If you want to trigger an action whenever the value of the
widget changes, you can connect to the QDateTimeEdit
dateTimeChanged()
signal.
If you want to create a widget for editing dates, you can use QDateEdit
. Similarly, you can use QTimeEdit
to create a widget for editing times only
PyQt QDateTimeEdit widget example
The following program uses the
class to create a widget for editing date & time:QDateTimeEdit
import sys
from PyQt6.QtWidgets import QApplication, QWidget, QDateTimeEdit, QLabel, QFormLayout
class MainWindow(QWidget):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.setWindowTitle('PyQt QDateTimeEdit')
self.setMinimumWidth(200)
layout = QFormLayout()
self.setLayout(layout)
self.datetime_edit = QDateTimeEdit(self, calendarPopup=True)
self.datetime_edit.dateTimeChanged.connect(self.update)
self.result_label = QLabel('', self)
layout.addRow('Date:', self.datetime_edit)
layout.addRow(self.result_label)
self.show()
def update(self):
value = self.datetime_edit.dateTime()
self.result_label.setText(value.toString("yyyy-MM-dd HH:mm"))
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
sys.exit(app.exec())
Code language: Python (python)
How it works.
First, create the
widget:QDateTimeEdit
self.datetime_edit = QDateTimeEdit(self, calendarPopup=True)
Code language: Python (python)
Second, connect the dateTimeChanged()
signal to the update()
method:
self.date_edit.dateTimeChanged.connect(self.update)
Code language: Python (python)
Third, create a
widget to display the value of the QLabel
widget once the editing is finished:date_edit
self.result_label = QLabel('', self)
Code language: Python (python)
Finally, define the update()
method that updates the label widget with the current value of the date & time entry:
def update(self):
value = self.datetime_edit.dateTime()
self.result_label.setText(value.toString("yyyy-MM-dd HH:mm"))
Code language: Python (python)
Output:
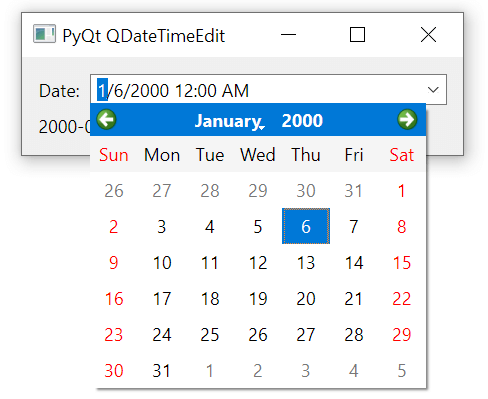
Summary
- Use the
to create a date and time entry widget.QDateTimeEdit
- Use the
dateTime()
method to get the current value of the
widgetQDateTimeEdit