Summary: in this tutorial, you’ll learn how to create a date entry widget using the PyQt QDateEdit
class.
Introduction to the PyQt QDateEdit widget
The QDateEdit
class provides you with a widget for editing dates based on QDateTimeEdit
class:
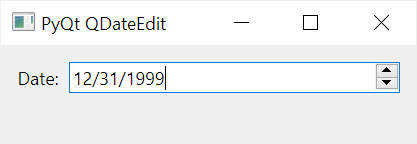
The QDateEdit
widget allows you to edit the date using the keyboard or up/down arrow keys to increase/decrease the date value.
In addition, you can use the left/right arrow key to move between the day, month, and year sections within the entry.
The QDateEdit
has the following useful properties:
Property | Description |
---|---|
date() | Return the date displayed by the widget. The return value has the type of . If you want to convert it to a Python datetime.date object, you can use the toPyDate() method of the class. |
minimumDate | Specify the earliest date that can be set by the user |
maximumDate | Specify the latest date that can be set by the user |
displayFormat | is a string that formats the date displayed in the widget |
The QDateEdit
emits the editingFinished
signal when the editing is finished.
PyQt QDateEdit widget example
The following program uses the QDateEdit
class to create a widget for editing date:
import sys
from PyQt6.QtWidgets import QApplication, QWidget, QDateEdit, QLabel, QFormLayout
from datetime import date
class MainWindow(QWidget):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.setWindowTitle('PyQt QDateEdit')
self.setMinimumWidth(200)
# create a grid layout
layout = QFormLayout()
self.setLayout(layout)
self.date_edit = QDateEdit(self)
self.date_edit.editingFinished.connect(self.update)
self.result_label = QLabel('', self)
layout.addRow('Date:', self.date_edit)
layout.addRow(self.result_label)
# show the window
self.show()
def update(self):
value = self.date_edit.date()
print(type(value))
self.result_label.setText(str(value.toPyDate()))
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
sys.exit(app.exec())
Code language: Python (python)
How it works.
First, create the QDateEdit
widget:
self.date_edit = QDateEdit(self)
Code language: Python (python)
Second, connect the editingFinished()
signal to the update()
method:
self.date_edit.editingFinished.connect(self.update)
Code language: Python (python)
Third, create a QLabel
widget to display the value of the date_edit
widget once the editing is finished:
self.result_label = QLabel('', self)
Code language: Python (python)
Finally, define the update()
method that updates the label widget with the current value of the date entry:
def update(self):
value = self.date_edit.date()
self.result_label.setText(str(value.toPyDate()))
Code language: Python (python)
Output:
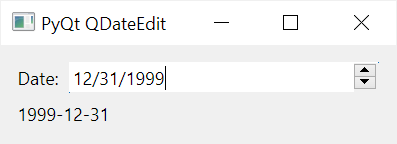
Summary
- Use the
QDateEdit
class to create a date entry widget. - Use the
date()
method to get the current value of theQDateEdit
widget - Connect to the
editingFinished
signal to trigger an action when editing is finished.