Summary: in this tutorial, you’ll learn how to use the PyQt QComboBox
to create a combobox widget.
Introduction to the PyQt QComboBox
A combobox provides you with a list of options so that you can select one of them. A combobox is also known as a dropdown or select widget.
To create a combobox, you use the QComboBox
class:
combobox = QCombobBox(self)
Code language: Python (python)
After creating a combobox, you need to populate its options by using:
addItem()
– takes a string label and a data value and appends it to the end of the list.insertItem()
– works like theaddItem()
method except that it takes an index for the first argument and adds the item at that index to the list.
Also, you can pass a list of options to the addItems()
or insertItems()
method at once.
To get the currently selected items, you can use one of the following methods:
currentData()
– returns the currently selected item.currentIndex()
– returns the index of the currently selected item.currentText()
– returns the text of the currently selected item.
Optionally, a combobox allows you to enter text if you set its editable property to True
.
The insertPolicy
property allows you to set whether the combobox should insert entered items in the list.
PyQt QComboBox example
The following example uses the QCombobox
class to create a combobox:
import sys
from PyQt6.QtWidgets import QApplication, QWidget, QRadioButton, QLabel, QVBoxLayout, QComboBox
from PyQt6.QtCore import Qt
class MainWindow(QWidget):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.setWindowTitle('PyQt QComboBox')
self.setMinimumWidth(300)
# create a grid layout
layout = QVBoxLayout()
self.setLayout(layout)
cb_label = QLabel('Please select a platform:', self)
# create a combobox
self.cb_platform = QComboBox(self)
self.cb_platform.addItem('Android')
self.cb_platform.addItem('iOS')
self.cb_platform.addItem('Windows')
self.cb_platform.activated.connect(self.update)
self.result_label = QLabel('', self)
layout.addWidget(cb_label)
layout.addWidget(self.cb_platform)
layout.addWidget(self.result_label)
# show the window
self.show()
def update(self):
self.result_label.setText(
f'You selected {self.cb_platform.currentText()}')
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
sys.exit(app.exec())
Code language: Python (python)
Output:
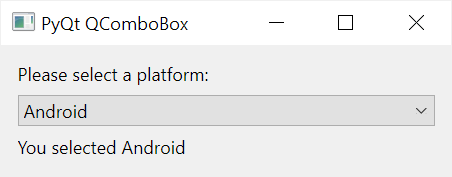
How it works.
First, create a combobox widget:
self.cb_platform = QComboBox(self)
Code language: Python (python)
Second, populate items by using the addItem()
method:
self.cb_platform.addItem('Android')
self.cb_platform.addItem('iOS')
self.cb_platform.addItem('Windows')
Code language: Python (python)
Third, connect the activated signal to the
method:self.update
()
self.cb_platform.activated.connect(self.update)
Code language: Python (python)
The combobox emits the activated signal when you change the item of the combobox.
Finally, define the update()
method that updates the text of the result_label
to the selected item of the combobox.
def update(self):
self.result_label.setText(
f'You selected {self.cb_platform.currentText()}')
Code language: Python (python)
Note that we use the currentText()
method to get the text of the currently selected item.
Summary
- Use PyQt
QComboBox
to create a combobox. - Use
addItem()
orinsertItem()
to add an item to the list of the combobox. - Connect to the
activated
signal to trigger an action when the selected item of a combobox changes.