Summary: in this tutorial, you’ll learn about how to use the Python issubset()
method to check if a set is a subset of another set.
Introduction to the Python issubset() method #
Suppose that you have two sets A and B. Set A is a subset of set B if all elements of A are also elements of B. Then, set B is a superset of set A.
The following Venn diagram illustrates that set A is a subset of the set B:
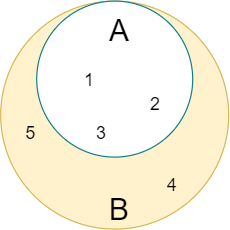
Set A and set B can be equal. If set A and set B are not equal, A is a proper subset of B.
In Python, you can use the Set issubset()
method to check if a set is a subset of another:
set_a.issubset(set_b)
Code language: CSS (css)
If the set_a
is a subset of the set_b
, the issubset()
method returns True
. Otherwise, it returns False
.
The following example uses the issubset()
method to check if the set_a
is a subset of the set_b
:
numbers = {1, 2, 3, 4, 5}
scores = {1, 2, 3}
print(scores.issubset(numbers))
Code language: PHP (php)
Output:
True
Code language: PHP (php)
By definition, a set is also a subset of itself. The following example returns True
:
numbers = {1, 2, 3, 4, 5}
print(numbers.issubset(numbers))
Code language: PHP (php)
Output:
True
Code language: PHP (php)
The following example returns False
because some elements in the numbers
set aren’t in the scores
set. In other words, the numbers
set is not a subset of the scores
set:
numbers = {1, 2, 3, 4, 5}
scores = {1, 2, 3}
print(numbers.issubset(scores))
Code language: PHP (php)
Output:
False
Code language: PHP (php)
Using subset operators #
Besides using the issubset()
method, you can use the subset operator (<=
) to check if a set is a subset of another set:
set_a <= set_b
The subset operator (<=
) returns True
if set_a
is a subset of the set_b
. Otherwise, it returns False
. For example:
numbers = {1, 2, 3, 4, 5}
scores = {1, 2, 3}
result = scores <= numbers
print(result) # True
result = numbers <= numbers
print(result) # True
Code language: PHP (php)
The proper subset operator (<
) check if the set_a
is a proper subset of the set_b
:
set_a < set_b
For example:
numbers = {1, 2, 3, 4, 5}
scores = {1, 2, 3}
result = scores < numbers
print(result) # True
result = numbers < numbers
print(result) # False
Code language: PHP (php)
In this example, the set numbers
is not a proper subset of itself, therefore, the <
operator returns False
.
Summary #
- Set
A
is a subset of setB
if all elements of the setA
are also elements of the setB
- Use Set
issubset()
method returnsTrue
if a set is a subset of another set. - Also, use the subset operator (<=) or the proper subset operator (<) to check if a set is a subset or proper subset of another set.